kintoneアプリ作成から kintone REST API をPHPのcURL関数で叩いてレコードを追加する方法のメモ。
kintoneアプリ作成
アプリ作成
“文字列(1行)”の入力フォームを3つ配置して氏名、電話番号、メールアドレスと設定します。(※フィールドコードは name / tel / email と設定)
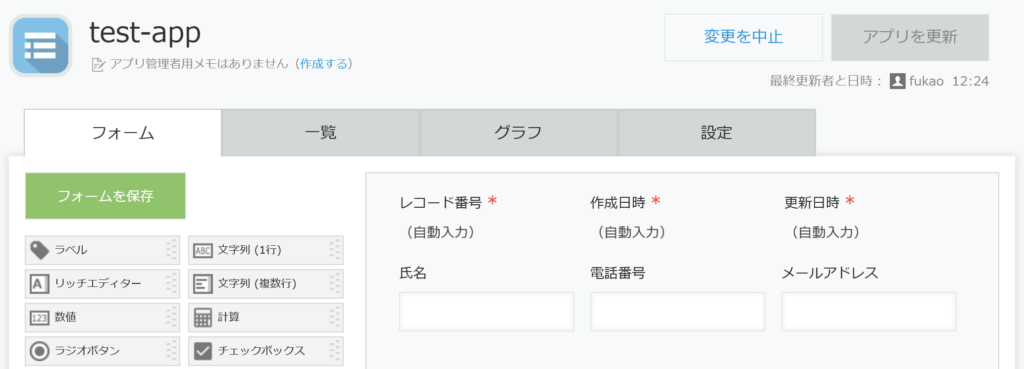
APIトークン生成
アプリ設定の「APIトークン」からAPIトークンを生成します。アクセス権は “レコード追加” のみにチェックをいれて保存します。
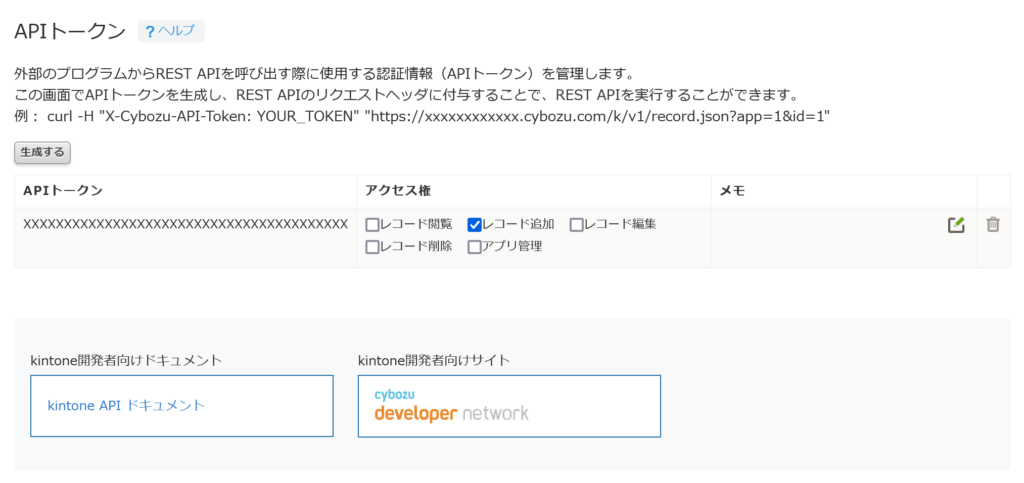
レコード追加処理
KINTONE関連設定
// KINTONE APIトークン
define('KINTONE_API_TOKEN', 'xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); //
// KINTONE アプリID
define('KINTONE_APP_ID', 1); //
// KINTONE サブドメイン
define('KINTONE_SUBDOMAIN', 'xxxxxxxxxxxx');
// KINTONE URL
define('KINTONE_URL', 'https://'.KINTONE_SUBDOMAIN.'.cybozu.com/k/v1/record.json');
ダミーデータ設定
$name = 'きんとーん てすと';
$tel = '000-0000-0000';
$email = 'example@example.com';
cURL送信内容設定
リクエストヘッダー
// リクエストヘッダー
$headers = [
'X-Cybozu-API-Token: ' . KINTONE_API_TOKEN,
'Content-Type: application/json'
];
POST内容
// POSTする内容(name, tel, email はフィールドコード)
$fields = [
'app' => KINTONE_APP_ID,
'record' =>
[
'name' => [
'value' => $name
],
'tel' => [
'value' => $tel
],
'email' => [
'value' => $email
]
]
];
$fields_json = json_encode($fields);
cURLセッション初期化から終了
curlセッション初期化
$ch = curl_init();
オプション設定
curl_setopt($ch, CURLOPT_URL, KINTONE_URL); // URL
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'POST'); // メソッド名
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); // ヘッダー
curl_setopt($ch, CURLOPT_POSTFIELDS, $fields_json); // POSTで送信するデータ
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // 返り値を文字列で返す(false にすると出力される)
curl実行
$response = curl_exec($ch);
curlセッション終了
curl_close($ch);
レコード追加サンプルコード
<?php
/**
* KINTONE関連設定
*/
// KINTONE APIトークン
define('KINTONE_API_TOKEN', 'xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); //
// KINTONE アプリID
define('KINTONE_APP_ID', 1); //
// KINTONE サブドメイン
define('KINTONE_SUBDOMAIN', 'xxxxxxxxxxxx');
// KINTONE URL
define('KINTONE_URL', 'https://'.KINTONE_SUBDOMAIN.'.cybozu.com/k/v1/record.json');
/**
* ダミーデータ設定
*/
$name = 'きんとーん てすと';
$tel = '000-0000-0000';
$email = 'example@example.com';
/**
* cURL送信内容設定
*/
// リクエストヘッダー
$headers = [
'X-Cybozu-API-Token: ' . KINTONE_API_TOKEN,
'Content-Type: application/json'
];
// POSTする内容(name, tel, email はフィールドコード)
$fields = [
'app' => KINTONE_APP_ID,
'record' =>
[
'name' => [
'value' => $name
],
'tel' => [
'value' => $tel
],
'email' => [
'value' => $email
]
]
];
$fields_json = json_encode($fields);
/**
* cURLセッション初期化から終了
*/
// curlセッション初期化
$ch = curl_init();
// オプション設定
curl_setopt($ch, CURLOPT_URL, KINTONE_URL); // URL
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'POST'); // メソッド名
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); // ヘッダー
curl_setopt($ch, CURLOPT_POSTFIELDS, $fields_json); // POSTで送信するデータ
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // 返り値を文字列で返す(false にすると出力される)
// curl実行
$response = curl_exec($ch);
// curlセッション終了
curl_close($ch);
exit;
動作環境情報
"エックスサーバー" スタンダード(旧X10) "PHP" 7.4.33 kintone スタンダードコース(開発者アカウント)
コメント