ShopifyのAPIを利用して商品リストや個別の商品情報をJSON形式のデータで取得することが出来ます。
Shopify API で商品リストJSONを取得する
下記サンプルは商品IDを指定して個別に商品情報を取得する場合、コレクションIDを指定して該当する商品のみのリストを取得する場合、条件を指定せず商品リストを取得する場合に利用出来ます。
各エンドポイント
個別の商品情報
https://{$shop_id}.myshopify.com/admin/api/{$api_version}/products/{$product_id}.json
コレクションIDに該当する商品リスト
https://{$shop_id}.myshopify.com/admin/api/{$api_version}/collections/{$collection_id}/products.json
条件指定しない商品リスト
https://{$shop_id}.myshopify.com/admin/api/{$api_version}/products.json
APIアクセススコープ
read_products を設定します。
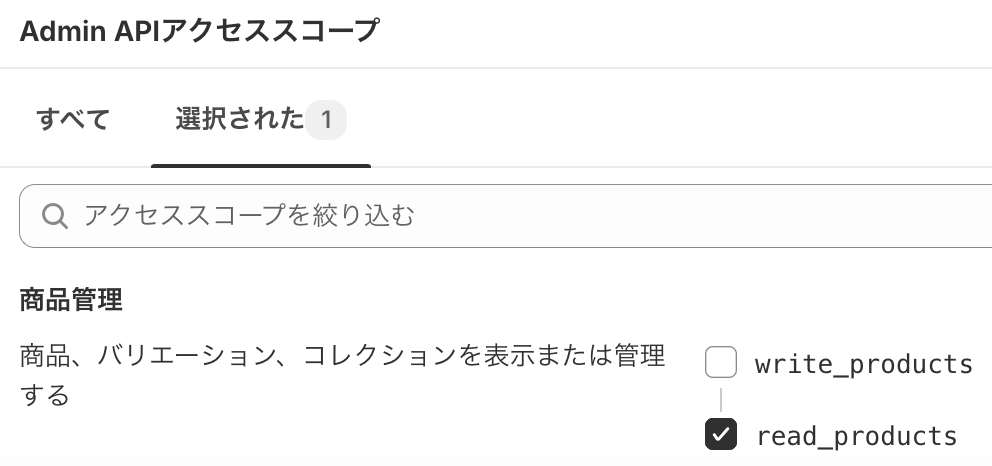
サンプルコード
<?php
// Shopify設定
$shop_id = "xxx123"; // ShopifyストアID(xxx123.myshopify.com の場合)
$accessToken = "shpat_********************************"; // APIアクセストークン
$api_version = "2024-04"; // APIバージョン
$product_id = $_GET['product_id'] ?? ''; // 商品のID
$collection_id = $_GET['collection_id'] ?? ''; // コレクションのID
// APIのURL
if ( $product_id ) {
// 商品IDを指定
$url = "https://{$shop_id}.myshopify.com/admin/api/{$api_version}/products/{$product_id}.json";
} else if ( $collection_id ) {
// コレクションIDを指定
$url = "https://{$shop_id}.myshopify.com/admin/api/{$api_version}/collections/{$collection_id}/products.json";
} else {
// ID指定なし
$url = "https://{$shop_id}.myshopify.com/admin/api/{$api_version}/products.json";
}
// cURLセッションの初期化
$ch = curl_init();
// cURLオプションの設定
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
"Content-Type: application/json",
"X-Shopify-Access-Token: {$accessToken}"
));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_VERBOSE, 0);
curl_setopt($ch, CURLOPT_HEADER, 0);
// APIリクエストの実行
$result = curl_exec($ch);
// エラーチェック
if(curl_errno($ch))
{
throw new Exception(curl_error($ch));
}
// cURLセッションの終了
curl_close($ch);
// 結果の表示
echo $result;
?>
コメント